Unity Openxr
This OpenXRInteractionFeature enables the use of Valve Index Controllers interaction profiles in OpenXR.
- Public const string featureId = 'com.unity.openxr.feature.input.valveindex' Field Value. Register action maps for this device with the OpenXR Runtime.
- MSBuild for Unity support. Support for MSBuild for Unity has been removed as of the 2.5.2 release, to align with Unity's new package guidance. Known issues OpenXR. There's currently a known issue with Holographic Remoting and OpenXR, where hand joints aren't consistently available.
Unity has developed a new plug-in framework called XR SDK that enables XR providers to integrate with the Unity engine and make full use of its features.
Inheritance
Inherited Members
Namespace: UnityEngine.XR.OpenXR.Features.Interactions
Syntax
Fields
aim
Constant for a pose interaction binding '.../input/aim/pose' OpenXR Input Binding.
Declaration
Field Value
Type | Description |
---|---|
String |
buttonA
Constant for a boolean interaction binding '.../input/a/click' OpenXR Input Binding.
Declaration
Field Value
Type | Description |
---|---|
String |
buttonATouch
Constant for a boolean interaction binding '.../input/a/touch' OpenXR Input Binding.
Declaration
Field Value
Type | Description |
---|---|
String |
buttonB
Constant for a boolean interaction binding '.../input/b/click' OpenXR Input Binding.
Declaration
Field Value
Type | Description |
---|---|
String |
buttonBTouch
Constant for a boolean interaction binding '.../input/b/touch' OpenXR Input Binding.
Declaration
Field Value
Type | Description |
---|---|
String |
featureId
The feature id string. This is used to give the feature a well known id for reference.
Declaration
Field Value
Type | Description |
---|---|
String |
grip
Constant for a pose interaction binding '.../input/grip/pose' OpenXR Input Binding.
Declaration
Field Value
Type | Description |
---|---|
String |
haptic
Constant for a haptic interaction binding '.../output/haptic' OpenXR Input Binding.
Declaration
Field Value
Type | Description |
---|---|
String |
profile
The interaction profile string used to reference the Valve Index Controller.
Declaration
Field Value
Type | Description |
---|---|
String |
squeeze
Constant for a float interaction binding '.../input/squeeze/value' OpenXR Input Binding.
Declaration
Field Value
Type | Description |
---|---|
String |
squeezeForce
Constant for a float interaction binding '.../input/squeeze/force' OpenXR Input Binding.
Declaration
Field Value
Type | Description |
---|---|
String |

system
Constant for a boolean interaction binding '.../input/system/click' OpenXR Input Binding.
Declaration
Field Value
Type | Description |
---|---|
String |
systemTouch
Constant for a boolean interaction binding '.../input/system/touch' OpenXR Input Binding.
Declaration
Field Value
Type | Description |
---|---|
String |
thumbstick
Constant for a Vector2 interaction binding '.../input/thumbstick' OpenXR Input Binding.
Declaration
Field Value
Type | Description |
---|---|
String |

thumbstickClick
Constant for a boolean interaction binding '.../input/thumbstick/click' OpenXR Input Binding.
Declaration
Field Value
Type | Description |
---|---|
String |
thumbstickTouch
Constant for a boolean interaction binding '.../input/thumbstick/touch' OpenXR Input Binding.
Declaration
Field Value
Type | Description |
---|---|
String |
trackpad
Constant for a Vector2 interaction binding '.../input/trackpad' OpenXR Input Binding.
Declaration
Field Value
Type | Description |
---|---|
String |
trackpadForce
Constant for a float interaction binding '.../input/trackpad/force' OpenXR Input Binding.
Declaration
Field Value
Type | Description |
---|---|
String |
trackpadTouch
Constant for a boolean interaction binding '.../input/trackpad/touch' OpenXR Input Binding.
Declaration
Field Value
Type | Description |
---|---|
String |
trigger
Constant for a float interaction binding '.../input/trigger/value' OpenXR Input Binding.
Declaration
Field Value
Type | Description |
---|---|
String |
triggerClick
Constant for a boolean interaction binding '.../input/trigger/click' OpenXR Input Binding.
Declaration
Field Value
Type | Description |
---|---|
String |
triggerTouch
Constant for a boolean interaction binding '.../input/trigger/touch' OpenXR Input Binding.
Declaration
Field Value
Type | Description |
---|---|
String |
Methods
RegisterActionMapsWithRuntime()
Register action maps for this device with the OpenXR Runtime.Called at runtime before Start.
Declaration
Overrides
RegisterDeviceLayout()
Registers the ValveIndexControllerProfile.ValveIndexController layout with the Input System.
Declaration
Overrides
UnregisterDeviceLayout()
Removes the ValveIndexControllerProfile.ValveIndexController layout from the Input System.
Declaration
Overrides
Caution
See this page on the new docs website.We've moved so we can provide you with a better docs experience. We will no longer be maintaing documentation on Github.Check out the new site to get started with MRTK in Unity!
XR SDK is Unity's new XR pipeline in Unity 2019.3 and beyond. In Unity 2019, it provides an alternative to the existing XR pipeline. In Unity 2020, it will become the only XR pipeline in Unity.
Prerequisites
To get started with the Mixed Reality Toolkit, follow the provided steps to add MRTK to a project.
Add XR SDK to a Unity project
Windows Mixed Reality, Oculus, and OpenXR are supported on XR SDK.
Required in Unity
OpenXR (Preview)
Important
OpenXR in Unity is only supported on Unity 2020.2 and higher.
Currently, it also only supports x64 and ARM64 builds.
- Follow the Using the Mixed Reality OpenXR Plugin for Unity guide, including the steps for configuring XR Plugin Management and Optimization to install the OpenXR plug-in to your project.
- Ensure that the following have successfully installed:
- XR Plugin Management
- OpenXR Plugin
- Mixed Reality OpenXR Plugin
Note
For the initial release of MRTK and OpenXR, only the HoloLens 2 articulated hands and Windows Mixed Reality motion controllers are natively supported. Support for additional hardware will be added in upcoming releases.
Windows Mixed Reality
- Go into Unity's Package Manager and install the Windows XR Plugin package, which adds support for Windows Mixed Reality on XR SDK. This will pull down a few dependency packages as well. Ensure the following all successfully installed:
- XR Plugin Management
- Windows XR Plugin
- XR Legacy Input Helpers
- Go to Edit > Project Settings.
- Click on the XR Plug-in Management tab in the Project Settings window.
- Go to the Universal Windows Platform settings and ensure Windows Mixed Reality is checked under Plug-in Providers.
- Ensure that Initialize XR on Startup is checked.
- (Required for in-editor HoloLens Remoting, otherwise optional) Go to the Standalone settings and ensure Windows Mixed Reality is checked under Plug-in Providers. Also ensure that Initialize XR on Startup is checked.
- (Optional) Click on the Windows Mixed Reality tab under XR Plug-in Management and create a custom settings profile to change the defaults. If the list of settings are already there, no profile needs to be created.
Required in MRTK
If using OpenXR, choose 'DefaultOpenXRConfigurationProfile' as the active profile or clone it to make customizations.
If using other XR runtimes in the XR Plug-in Management configuration, like Windows Mixed Reality or Oculus, choose 'DefaultXRSDKConfigurationProfile' as the active profile or clone it to make customizations.
These profiles are set up with the correct systems and providers, where needed.
To migrate an existing profile to XR SDK, the following services and data providers should be updated:
Camera
Unity Openxr Steam
From WindowsMixedReality.WindowsMixedRealityCameraSettings
to
OpenXR | Windows Mixed Reality |
---|---|
GenericXRSDKCameraSettings | XRSDK.WindowsMixedReality.WindowsMixedRealityCameraSettings andGenericXRSDKCameraSettings |
Input
From WindowsMixedReality.Input.WindowsMixedRealityDeviceManager
to
OpenXR | Windows Mixed Reality |
---|---|
OpenXRDeviceManager | XRSDK.WindowsMixedReality.WindowsMixedRealityDeviceManager |
OpenXR:
Windows Mixed Reality:
Xr Plugin Unity
Boundary
From MixedRealityBoundarySystem
to
OpenXR | Windows Mixed Reality |
---|---|
XRSDKBoundarySystem | XRSDKBoundarySystem |
Spatial awareness
From WindowsMixedReality.SpatialAwareness.WindowsMixedRealitySpatialMeshObserver
to
OpenXR | Windows Mixed Reality |
---|---|
In progress | XRSDK.WindowsMixedReality.WindowsMixedRealitySpatialMeshObserver |
Controller mappings
If using custom controller mapping profiles, open one of them and run the Mixed Reality Toolkit -> Utilities -> Update -> Controller Mapping Profiles menu item to ensure the new XR SDK controller types are defined.
See also
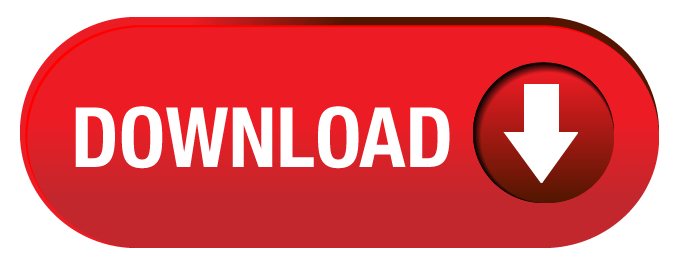